Download and install python
Go to python.org official website and download the latest version.
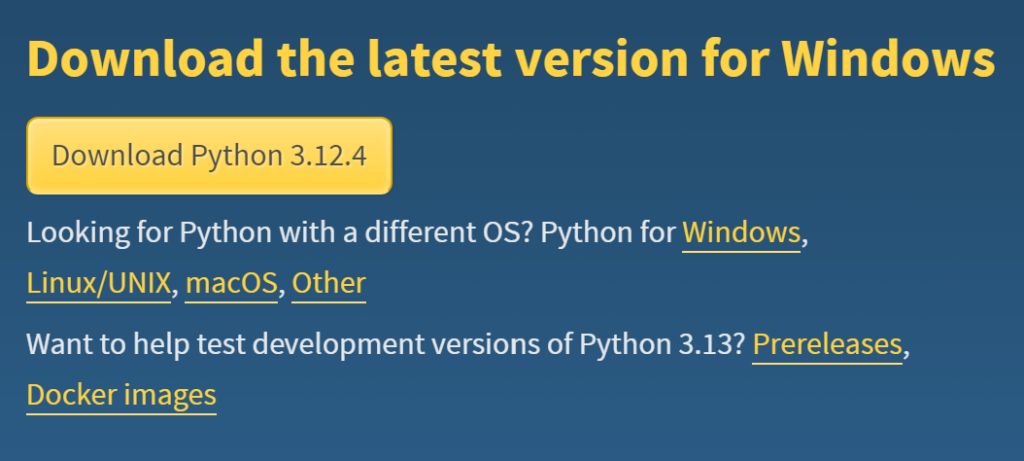
Make sure you’ve selected the checkbox for adding the Python path to the Environment Variable.
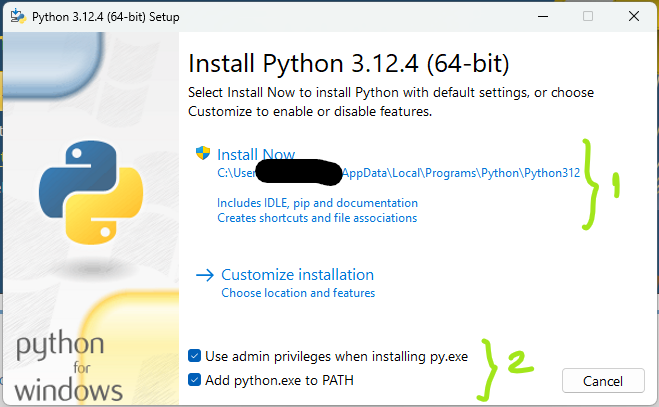
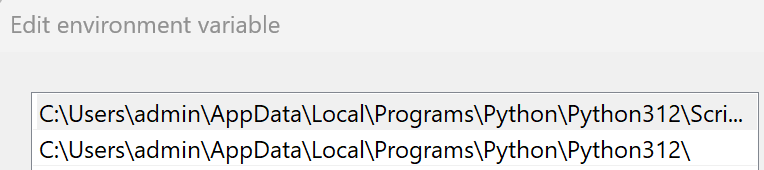
After you install the latest version, open CMD and type the following python –version.

Congrats, Python was successfully installed!
PIP
This is the standard package manager for Python. It allows you to install and manage additional packages that are not part of the Python standard library.
It comes with python and you can locate it in the Scripts folder C:\Users\{{username}}\AppData\Local\Programs\Python\Python312\Scripts
Install Selenium for Python
Open CMD as Administrator and type pip install selenium
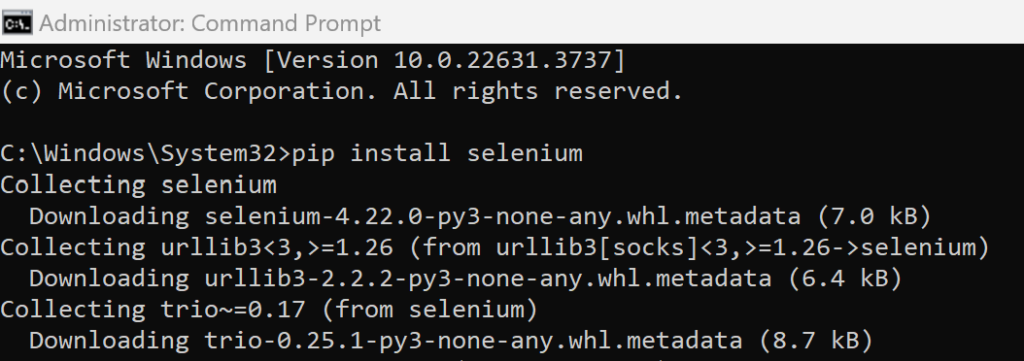
pip –help to check all available options.
pip show <package name>
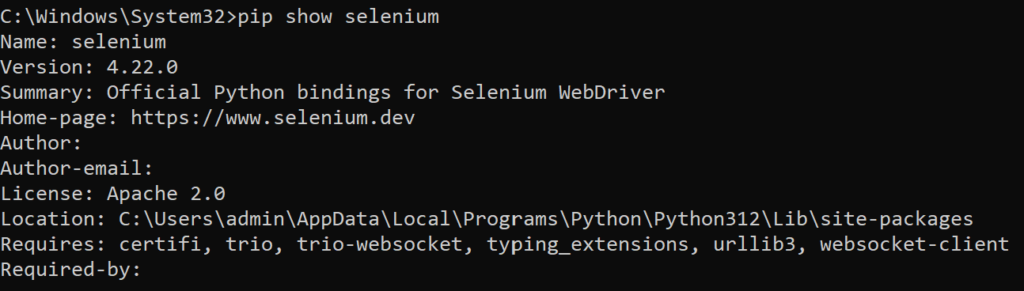
Install PyCharm
Download and install the community edition as this is the free version.
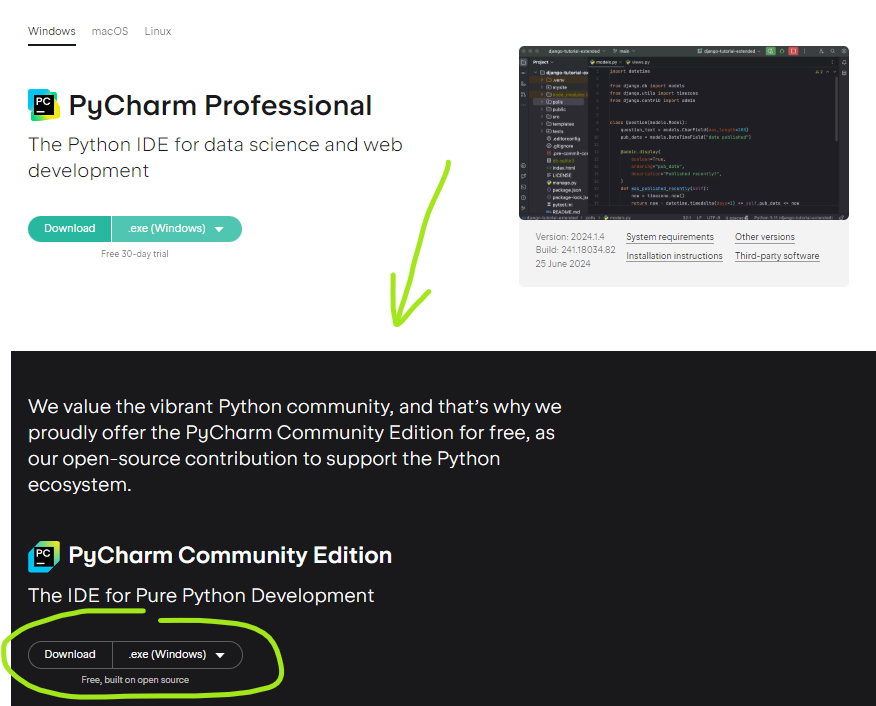
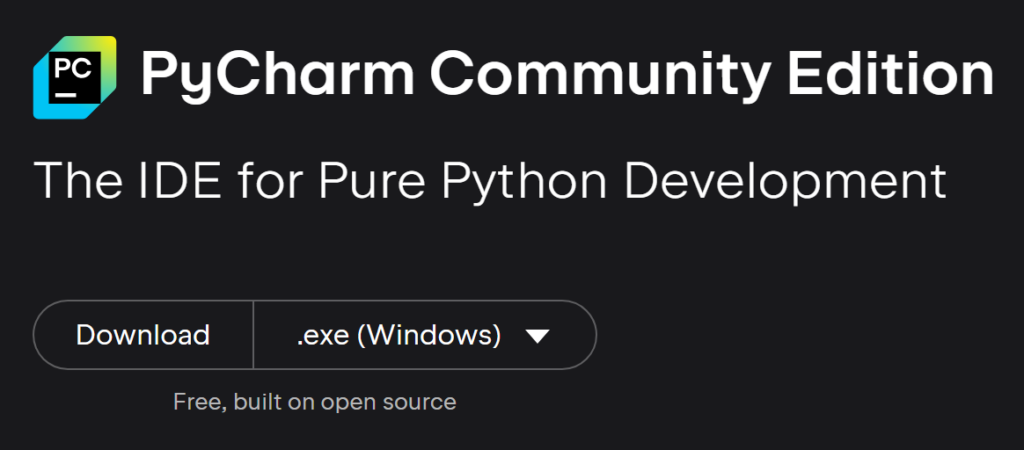
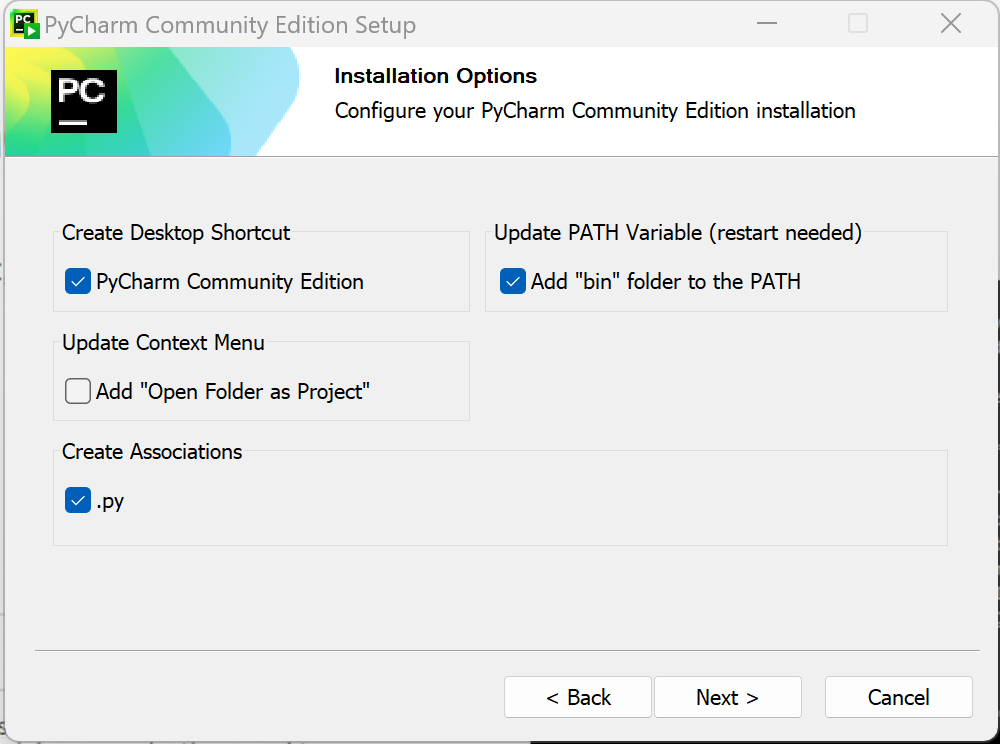
Open the PyCharm and create a new project. Go to Interpreter Settings and set the
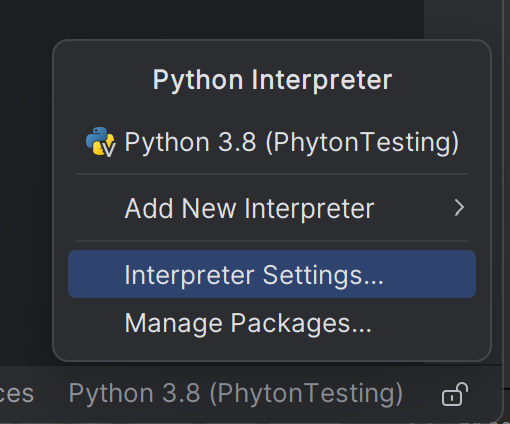
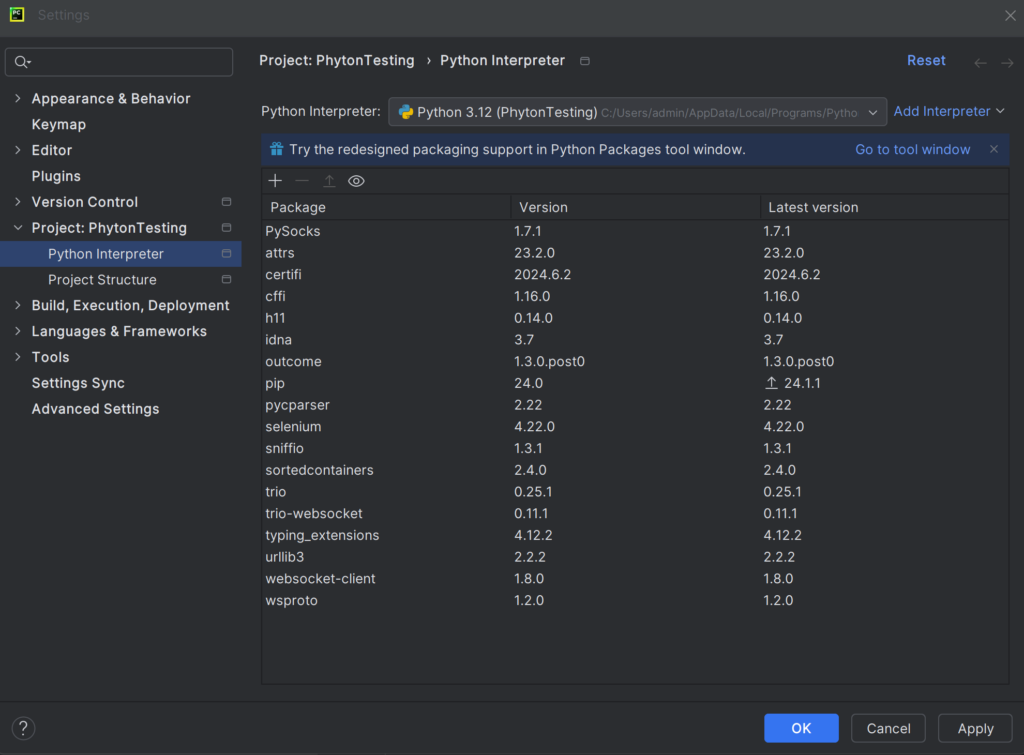
My first Python program
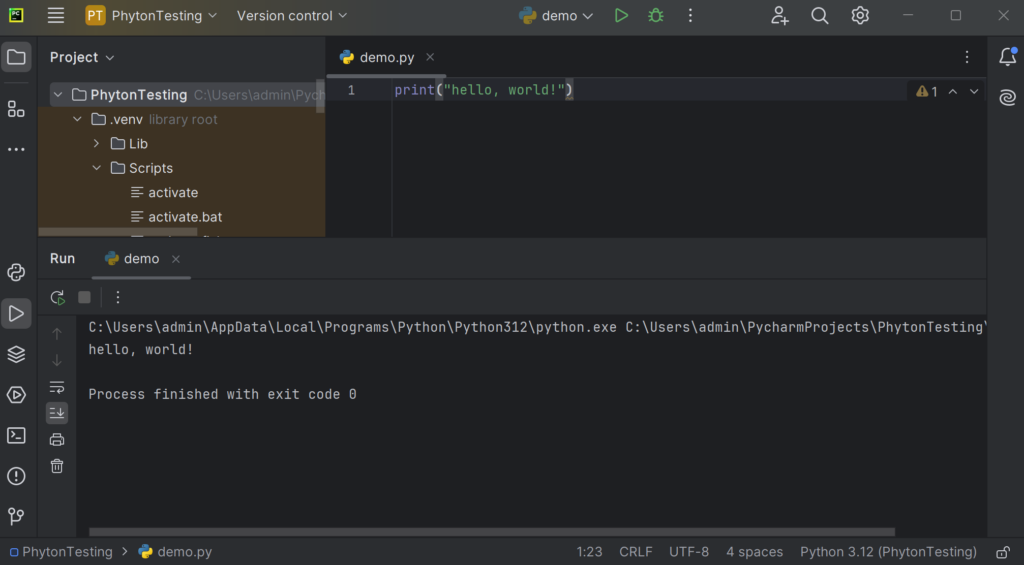
Python Data Types
Download ChromeDriver
https://sites.google.com/chromium.org/driver/downloads?authuser=0
https://googlechromelabs.github.io/chrome-for-testing
https://storage.googleapis.com/chrome-for-testing-public/126.0.6478.126/win64/chrome-win64.zip
PyTest Testing Framework
How to install PyTest
Go to CMD and type the following line
pip install pytest
To check the installed version, write
pytest --version
PyTest Framework Standards
- PyTest files should start with test_ or end with _test
- PyTest methods should start with test_
- Any code should be wrapped in a method
Run PyTest from PyCharm
In PyCharm, create a new project, then create a new package (call it pytestsDemo). Inside create a new file called test_demo1.py. Paste this code:
def test_firstProgram():
print("Hello")
To execute it, go to Edit Configuration …
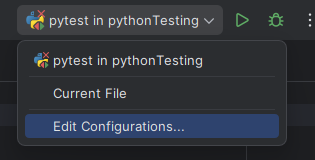
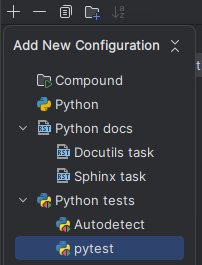
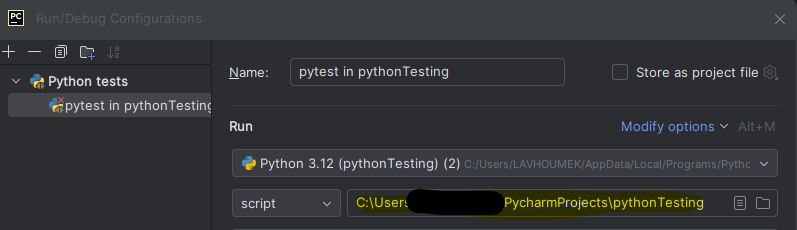
The test results should look like this
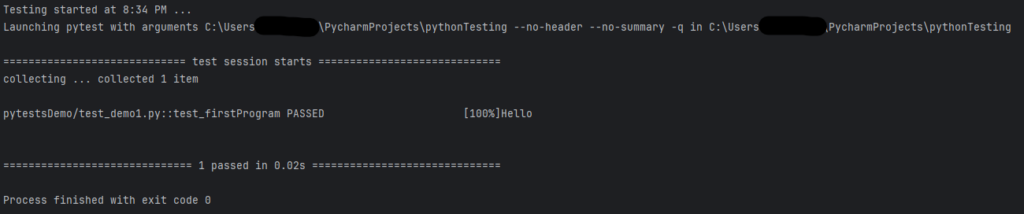
Examples
def test_firstProgram():
print("Hello")
def test_secondProgram():
print("Hello 2")
def test_secondProgram():
msg = "Hello"
assert msg == "Hi", "Test failed because the 2 strings don't match"
Run PyTest from CMD
Open CMD, go to the package location (cd absolute path) and run
py.test -v -s
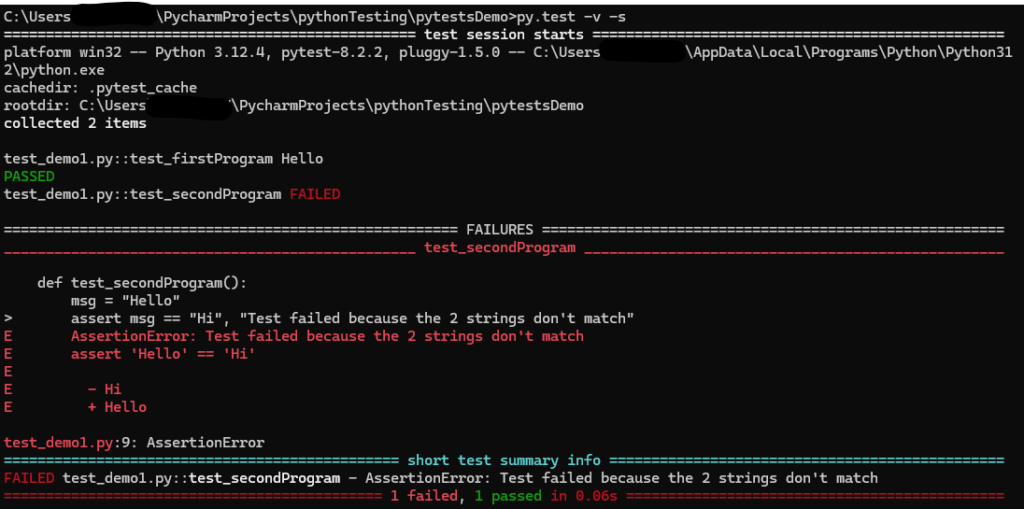
Use the -k option to run tests only for the methods that contain the specific keyword (in the example below I used “loginPage”)
py.test -k loginPage -v -s
Another option is to group tests with PyTest mark.
import pytest
@pytest.mark.smoke
def test_firstProgram():
print("Hello")
def test_secondProgram():
msg = "Hello"
assert msg == "Hi", "Test failed because the 2 strings don't match"
@pytest.mark.smoke
def test_thirdProgram():
i = 1
j = 2
assert i+j == 3, "The sum of the 2 numbers doesn't match"
@pytest.mark.smoke, @pytest.mark.skip, @pytest.mark.xfail
PyTest Fixture
A fixture in PyTest is a function that provides data, objects, or resources to test functions.
import pytest
@pytest.fixture
def input_value():
return 39
def test_divisible_by_3(input_value):
assert input_value % 3 == 0
conftest.py
Generate HTML reports
To generate the reports for all your tests you need to install pytest-html
pip install pytest-html
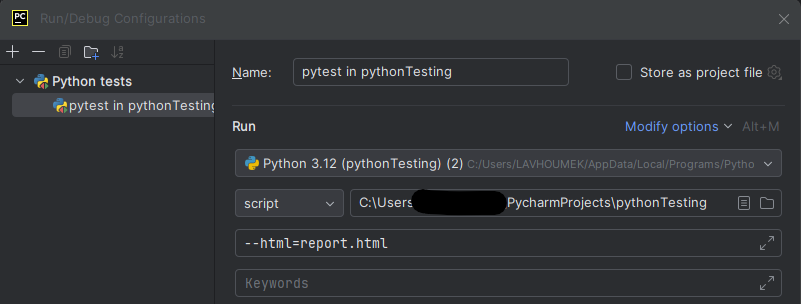
--html=report.html
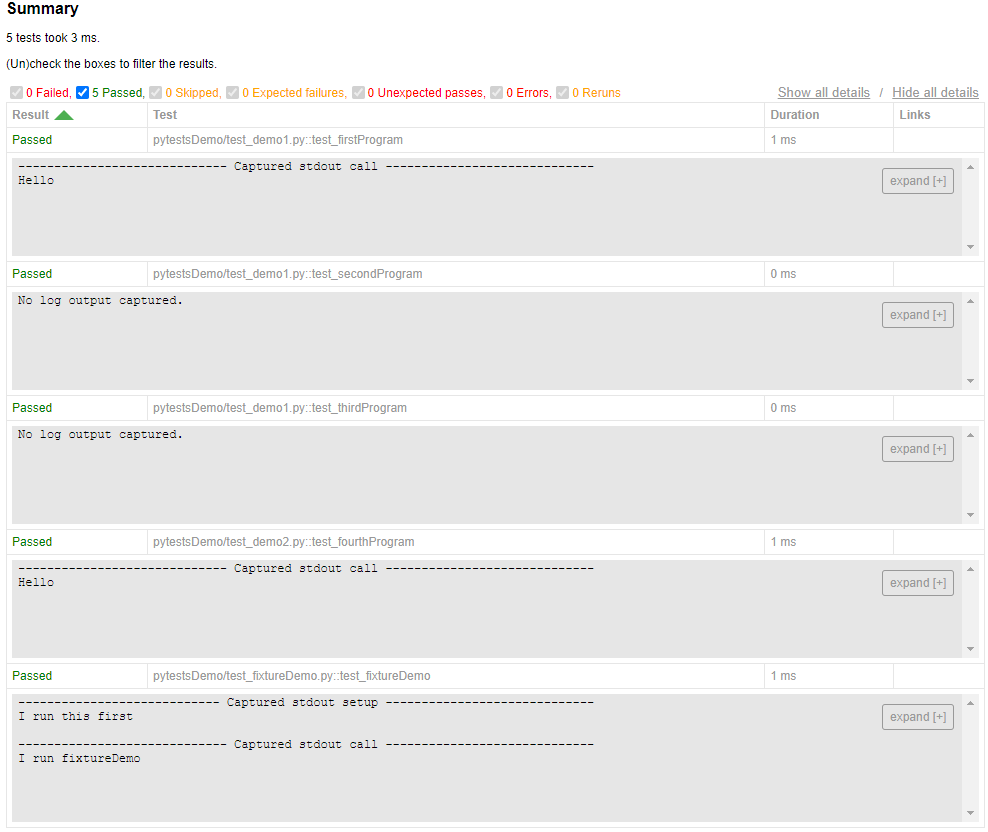
Logging in Python Tests
Types
- Debug
- Info
- Warning
- Error
- Critical
import inspect
import logging
class BaseClass:
def getLogger(self):
loggerName = inspect.stack()[1][3]
# logger = logging.getLogger(__name__)
logger = logging.getLogger(loggerName)
fileHandler = logging.FileHandler('logfile.log')
formatter = logging.Formatter("%(asctime)s: [%(levelname)s] %(name)s: %(message)s")
fileHandler.setFormatter(formatter)
logger.addHandler(fileHandler)
# set the logging level
logger.setLevel(logging.INFO)
return logger