Mocha is a popular JavaScript testing framework that is widely used for automated testing with Selenium. It provides a structured way to write and organize test cases, making it easier to manage and execute automated tests for web applications.
Why Mocha
Here are some key points about using Mocha for automated testing with Selenium:
- Test Structure: Mocha follows the Behavior-Driven Development (BDD) style of writing tests, using a descriptive and readable syntax. Tests are organized into “describe” blocks (for test suites) and “it” blocks (for individual test cases).
- Assertions: Mocha is often used in conjunction with assertion libraries like Chai, which provide a rich set of assertions for verifying the expected behavior of web applications.
- Asynchronous Testing: Mocha supports asynchronous testing, which is essential when working with Selenium, as many browser interactions are asynchronous in nature.
- Test Hooks: Mocha provides hooks (like before, after, beforeEach, and afterEach) that allow you to set up and tear down test environments, making it easier to manage test setup and cleanup.
- Parallel Test Execution: Mocha supports parallel test execution, which can significantly reduce the overall test execution time, especially when running tests on cloud-based Selenium grids like LambdaTest or BrowserStack.
- Reporting: Mocha generates readable test reports in the console, making it easier to identify and debug failing tests. It can also be integrated with various reporting tools for more comprehensive reporting.
- Integration with Selenium: Mocha can be easily integrated with Selenium WebDriver, allowing you to write automated tests that interact with web browsers using JavaScript.
By combining Mocha with Selenium WebDriver, developers and testers can create robust and maintainable automated test suites for web applications, ensuring consistent and reliable testing across different browsers and platforms.
How to install Mocha on your project
Open the terminal and type in the following code:
npm i mocha
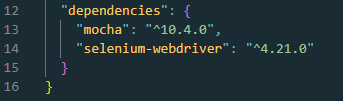
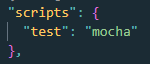
First Test
In Visual Studio Code create a new file under the folder tests. Call the file mochaTest.js
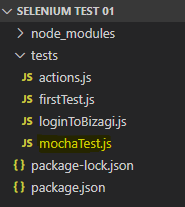
const { Builder, Browser, By, Key, until } = require("selenium-webdriver");
describe("Run the file mochaTest.js", function () {
this.timeout(0);
let driver;
it("implicit await", async function () {
driver = await new Builder().forBrowser(Browser.CHROME).build();
await driver.get("https://www.google.com/ncr");
});
// hooks afterAll, afterEach etc
this.afterEach(async function () {
await driver.quit();
});
});
npm test .\tests\mochaTest.js
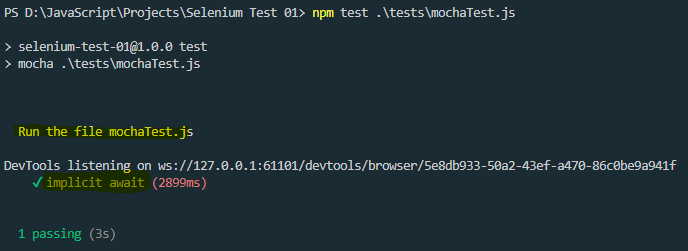